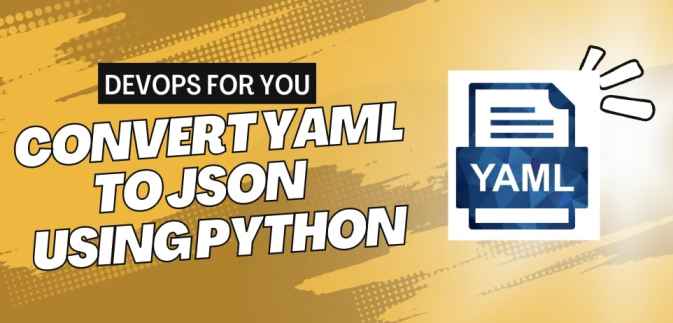
This article will explore how to convert YAML to JSON using Python. YAML and JSON are popular data serialization formats, often used in configuration files and data exchange between languages with different data structures. While they serve similar purposes, sometimes we need to convert between these formats. Python makes this conversion straightforward and efficient with the help of libraries.
Step-by-Step Conversion Process
Step 1: Install the PyYAML Library
Before we begin, ensure that you have Python installed and the PyYAML library. You can install the PyYAML library using pip:
pip install PyYAML
Step 2: Write a Function to Convert YAML to JSON
To convert a YAML string to a JSON string, you can use the following Python function:
import yaml
import json
def yaml_to_json(yaml_str):
yaml_data = yaml.safe_load(yaml_str)
json_str = json.dumps(yaml_data, indent=2)
return json_str
# Sample YAML string
yaml_str = """
name: John Doe
age: 30
is_student: false
subjects:
- Math
- Science
- History
"""
json_str = yaml_to_json(yaml_str)
print(json_str)
This function first parses the YAML string using the safe_load
function from the yaml
module. Then, it converts the resulting Python object to a JSON string using the dumps
function from the json
module.
Step 3: Write a Function to Convert a YAML File to a JSON File
If you have a YAML file and want to convert it to a JSON file, you can use the following Python script:
import yaml
import json
def yaml_file_to_json_file(yaml_file_path, json_file_path):
with open(yaml_file_path, 'r') as yaml_file:
yaml_data = yaml.safe_load(yaml_file)
with open(json_file_path, 'w') as json_file:
json.dump(yaml_data, json_file, indent=2)
yaml_file_path = 'input.yaml'
json_file_path = 'output.json'
yaml_file_to_json_file(yaml_file_path, json_file_path)
This script reads a YAML file named input.yaml
, converts its contents to JSON, and writes the result to a new file named output.json
. You can change the file names as needed.
Benefits of Converting YAML to JSON
There are several benefits to converting YAML to JSON:
- Compatibility: Some systems and libraries only support JSON, so converting YAML to JSON can help ensure compatibility.
- Verbosity: JSON is more compact than YAML, which can result in smaller file sizes and reduced bandwidth usage when transmitting data.
- Parsing speed: In some cases, parsing JSON can be faster than parsing YAML, especially for large data structures.
In summary, converting YAML to JSON using Python is simple and efficient. Python’s built-in json
module and the PyYAML
the library makes the conversion process straightforward. Converting between YAML and JSON can provide compatibility, reduced file size, and improved parsing speed in certain cases. With the examples provided in this article, you can easily perform the conversion for both strings and files.
Follow us on LinkedIn for updates!