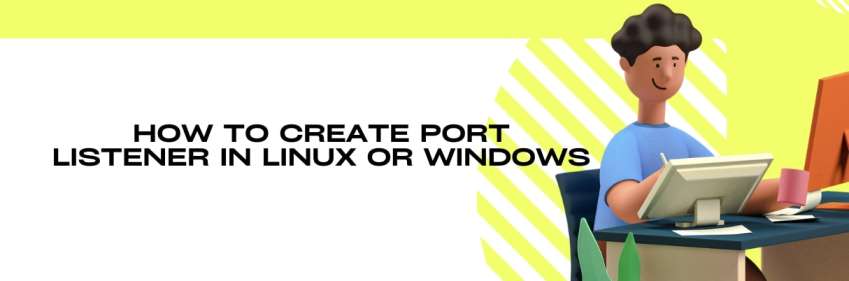
Port listener is a program or process that listens for incoming connections on a specific port. When a connection request is received on that port, the listener accepts the connection and establishes a two-way communication channel with the client that made the request.
Port listeners are commonly used for various purposes, such as:
- Server applications that need to receive data or requests from clients on a specific port.
- Network monitoring tools that capture and analyze network traffic on specific ports.
- Security tools that monitor incoming connections and detect potential attacks or intrusion attempts on specific ports.
- Debugging tools that allow developers to inspect the data exchanged between clients and servers on specific ports.
Port listeners can be implemented in many programming languages, including Python, Once a connection is established, the listener can then handle incoming data or requests according to its specific purpose or functionality.
Step-by-step instructions on how to create a port listener
Using Netcat (nc)
- Open a terminal on your Linux machine.
- Type the following command and press Enter:
nc -v -l <port number>
. - Replace
<port number>
with the port number, you want to listen on. For example, if you want to listen on port 8080, the command would be
devops@DevOpsForU:~$ nc -v -l 8080
- The terminal will now be waiting for incoming connections. You can leave the terminal open and it will continue to listen.
- To test the port listener, open another terminal on the same or different machine and run the following command:
telnet <ip address or hostname> <port number>
. - Replace
<ip address or hostname>
with the IP address or hostname of the machine running the listener (if on the same machine, you can uselocalhost
or127.0.0.1
), and<port number>
with the port number, you specified earlier. For example, if the listener is running on a machine with IP address 192.168.64.4 and port 8080, the command would betelnet 192.168.64.4 8080
.
devopsforu@public:~$ telnet 192.168.64.4 8080
Trying 192.168.64.4...
Connected to 192.168.64.4.
Escape character is '^]'.
- If the listener is working correctly, the terminal running the listener will display the connection information and any data sent from the client.
Using Socat
- Open a terminal on your Linux.
- Type the following command and press Enter: socat -v tcp-listen:<port number>,reuseaddr,fork
- Replace
<port number>
with the port number. For example, if you want to listen on port 8081, the command would besocat -v tcp-listen:8081,reuseaddr,fork -
devops@DevOpsForU:~$ socat -v tcp-listen:8081,reuseaddr,fork -
- The terminal will now be waiting for incoming connections on the specified port. You can leave the terminal open and it will continue to listen in the background.
- To test the port listener, open another terminal window on the same machine or use a different machine and run the following command:
telnet <ip address or hostname> <port number>
. - Replace
<ip address or hostname>
with the IP address or hostname of the machine running the listener (if on the same machine, you can uselocalhost
or127.0.0.1
), and<port number>
with the port number, you specified earlier. For example, if the listener is running on a machine with IP address 192.168.64.4 and port 8081, the command would betelnet 192.168.64.4 8081
.
devopsforu@public:~$ telnet 192.168.64.4 8081
Trying 192.168.64.4...
Connected to 192.168.64.4.
Escape character is '^]'.
- If the listener is working correctly, the terminal running the listener will display the connection information and any data sent from the client.
Create a Port Listener in Python
To create a port listener in Python, you can use the socket
module. Here is an example of Python code to create a port listener:
devops@DevOpsForU:~$ vi port_listener.py
import socket
HOST = '' # Listen on all network interfaces
PORT = 8080 # Port number to listen on
# Create a socket object
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
# Bind the socket to a specific network interface and port number
s.bind((HOST, PORT))
print(f"Listening on port {PORT}...")
# Listen for incoming connections
s.listen()
# Accept incoming connections
conn, addr = s.accept()
print(f"Connected by {addr}")
# Receive data from the client
while True:
data = conn.recv(1024)
if not data:
break
print(f"Received data: {data.decode('utf-8').strip()}")
How to Run the python Port Listener code
- Open a terminal and navigate to the directory where you have saved the Python code file.
- Run the Python code by typing
python3 port_listener.py
and pressing Enter.
devops@DevOpsForU:~$ python3 port_listener.py
devops@DevOpsForU:~$ python3 port_listener.py
Listening on port 8080...
Connected by ('192.168.64.1', 56057)
Received data: hello
Conclusion
In conclusion, a port listener is a program that listens for incoming network connections on a specific network port. Port listeners are commonly used in network programming for various purposes, such as server applications, network monitoring tools, security tools, and debugging tools.
In Linux, you can create a port listener using various tools and programming languages such as nc
, socat
, and Python’s socket
module. These tools use low-level network APIs to manage the underlying network connections, and once a connection is established, the listener can handle incoming data or requests according to its specific purpose or functionality.
It’s important to use caution when opening network ports and ensure that you have proper security measures in place, such as firewalls and access controls, to protect your system from unauthorized access and potential security threats.
Frequently Asked Questions (FAQ)
Que 1: What is a port listener in networking?
Ans: Port listener is a program or process that listens for incoming network connections on a specific network port. It accepts incoming connection requests and establishes a two-way communication channel with the client.
Que 2: What are some common uses of port listeners?
Ans: Port listeners are commonly used in server applications, network monitoring tools, security tools, and debugging tools. They can be used to receive data or requests from clients on a specific port, capture and analyze network traffic on specific ports, monitor incoming connections, and detect potential attacks or intrusion attempts on specific ports.
Que 3: How can I create a port listener in Linux?
Ans: You can create a port listener in Linux using various tools such as nc
, socat
, and Python’s socket
module. These tools use low-level network APIs to manage the underlying network connections and handle incoming data or requests according to their specific purpose or functionality.
Que 4: Is it safe to use a port listener?
Ans: Using a port listener can be safe as long as you have proper security measures in place, such as firewalls and access controls. It’s important to be cautious when opening network ports and ensure that you are only allowing authorized access to your system.
Que 5: How can I test a port listener?
Ans: You can test a port listener by using a client tool such as telnet
or netcat
to connect to the server on the specified port and send data. This will allow you to verify that the listener is functioning properly and receiving data from the client.
This article is created based on experience but If you discover any corrections or enhancements, please write a comment in the comment section or email us at contribute@devopsforu.com. You can also reach out to us from Contact-Us Page.
Follow us on LinkedIn for updates!